HTML DOM Tutorial
The HTML Document Object Model
(HTML DOM) defines a standard way for accessing and manipulating HTML
documents.
The DOM presents an HTML
document as a tree-structure (a node tree), with elements, attributes, and
text.
What is the DOM?
The DOM is a W3C (World Wide Web
Consortium) standard.
The DOM defines a standard for
accessing documents like HTML and XML:
"The W3C Document Object
Model (DOM) is a platform and language-neutral interface that allows programs
and scripts to dynamically access and update the content, structure, and style
of a document."
The DOM is separated into 3
different parts / levels:
- Core DOM -
standard model for any structured document
- XML DOM -
standard model for XML documents
- HTML DOM -
standard model for HTML documents
The DOM defines the objects and
properties of all document elements, and the methods (interface) to
access them.
What is the XML DOM?
The XML DOM defines the objects
and properties of all XML elements, and the methods (interface) to
access them.
If you want to study the XML DOM,
find the XML DOM tutorial on our
homepage.
What is the HTML DOM?
The HTML DOM is:
- A standard
object model for HTML
- A standard
programming interface for HTML
- Platform- and
language-independent
- A W3C standard
The HTML DOM defines the objects
and properties of all HTML elements, and the methods (interface) to
access them.
In other words:
The HTML DOM is a standard for how
to get, change, add, or delete HTML elements.
HTML DOM Nodes
In the DOM, everything in an HTML
document is a node.
Nodes
According to the DOM, everything
in an HTML document is a node.
The DOM says:
- The entire document is a
document node
- Every HTML tag is an element
node
- The text in the HTML elements
are text nodes
- Every HTML attribute is an
attribute node
- Comments are comment nodes
DOM Example
Look at the following HTML
document:
<html> <head> <title>DOM Tutorial</title> </head> <body> <h1>DOM Lesson one</h1> <p>Hello world!</p> </body> </html> |
The root node in the HTML above is
<html>. All other nodes in the document are contained within
<html>.
The <html> node has two
child nodes; <head> and <body>.
The <head> node holds a
<title> node. The <body> node holds a <h1> and <p>
node.
Text is Always Stored in Text Nodes
A common error in DOM processing
is to expect an element node to contain text.
However, the text of an element
node is stored in a text node.
In this example: <title>DOM
Tutorial</title>, the element node <title>, holds a text node
with the value "DOM Tutorial".
"DOM Tutorial" is not
the value of the <title> element!
However, in the HTML DOM the value
of the text node can be accessed by the innerHTML property.
You can read more about the
innerHTML property in a later chapter.
HTML DOM Node Tree
The HTML DOM views a HTML document
as a node-tree.
All the nodes in a node tree have
relationships to each other.
The HTML DOM Node Tree (Document Tree)
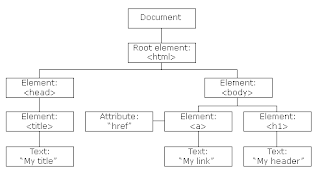
The HTML DOM views a HTML document
as a tree-structure. The tree structure is called a node-tree.
All nodes can be accessed through
the tree. Their contents can be modified or deleted, and new elements can be
created.
The node tree below shows the set
of nodes, and the connections between them. The tree starts at the root node
and branches out to the text nodes at the lowest level of the tree:
Node Parents, Children, and Siblings
The nodes in the node tree have a
hierarchical relationship to each other.
The terms parent, child, and
sibling are used to describe the relationships. Parent nodes have children.
Children on the same level are called siblings (brothers or sisters).
- In a node tree,
the top node is called the root
- Every node,
except the root, has exactly one parent node
- A node can have
any number of children
- A leaf is a
node with no children
- Siblings are
nodes with the same parent
Look at the following HTML
fragment:
<html> <head> <title>DOM Tutorial</title> </head> <body> <h1>DOM Lesson one</h1> <p>Hello world!</p> </body> </html> |
In the HTML above, every node
except for the document node has a parent node:
- The
<html> node has no parent node; the root node
- The parent node
of the <head> and <body> nodes is the <html> node
- The parent node
of the "Hello world!" text node is the <p> node
Most element nodes have child
nodes:
- The
<html> node has two child nodes; <head> and <body>
- The
<head> node has one child node; the <title> node
- The
<title> node also has one child node; the text node "DOM
Tutorial"
- The <h1>
and <p> nodes are siblings, and both child nodes of <body>
HTML DOM Properties and
Methods
Properties and methods define the
programming interface to the HTML DOM.
Programming Interface
The DOM models HTML as a set of
node objects. The nodes can be accessed with JavaScript or other programming
languages. In this tutorial we use JavaScript.
The programming interface to the
DOM is defined by a set standard properties and methods.
Properties are often referred to as something that is
(i.e. nodename is "p").
Methods are often referred to as something that is
done (i.e. delete "p").
HTML DOM Properties
These are some typical DOM
properties:
- x.innerHTML -
the inner text value of x (a HTML element)
- x.nodeName -
the name of x
- x.nodeValue -
the value of x
- x.parentNode -
the parent node of x
- x.childNodes -
the child nodes of x
- x.attributes -
the attributes nodes of x
Note: In the list above, x is a node object
(HTML element).
HTML DOM Methods
- x.getElementByID(id)
- get the element with a specified id
- x.getElementsByTagName(name)
- get all elements with a specified tag name
- x.appendChild(node)
- insert a child node to x
- x.removeChild(node)
- remove a child node from x
Note: In the list above, x is a node object
(HTML element).
innerHTML
The easiest way to get or modify
the content of an element is by using the innerHTML property.
innerHTML is not a part of the W3C
DOM specification. However, it is supported by all major browsers.
The innerHTML property is useful
for returning or replacing the content of HTML elements (including <html>
and <body>), it can also be used to view the source of a page that has
been dynamically modified.
Example
The JavaScript code to get the
text from a <p> element with the id "intro" in a HTML document:
txt=document.getElementById("intro").innerHTML
After the execution of the
statement, txt will hold the value "Hello World!"
Explained:
- document - the current
HTML document
- getElementById("intro") - the
<p> element with the id "intro"
- innerHTML - the inner
text of the HTML element
In the example above,
getElementById is a method, while innerHMTL is a property.
childNodes and nodeValue
The W3C DOM specified way of
getting to the content of an element works like this:
The JavaScript code to get the
text from a <p> element with the id "intro" in a HTML document:
txt=document.getElementById("intro").childNodes[0].nodeValue
After the execution of the
statement, txt will hold the value "Hello World!"
Explained:
- document - the current
HTML document
- getElementById("intro") - the
<p> element with the id "intro"
- childNodes[0] - the first
child of the <p> element (the text node)
- nodeValue - the value of
the node (the text itself)
In the example above,
getElementById is a method, while childNodes and nodeValue are properties.
In this tutorial we will mostly
use the innerHTML property. However, learning the method above is useful for
understanding the tree structure of the DOM and dealing with XML files.
HTML DOM Access Nodes
With the DOM, you can access every
node in an HTML document.
Accessing Nodes
You can access a node in three
ways:
1. By using the getElementById()
method
2. By using the
getElementsByTagName() method
3. By navigating the node tree,
using the node relationships.
The getElementById() Method
The getElementById() method
returns the element with the specified ID:
Syntax
document.getElementById("someID"); |
Note: The getElementById() method doesn't work
in XML.
The getElementsByTagName() Method
getElementsByTagName() returns all
elements with a specified tag name.
Syntax
node.getElementsByTagName("tagname"); |
Example 1
The following example returns a
nodeList of all <p> elements in the document:
document.getElementsByTagName("p"); |
Example 2
The following example returns a
nodeList of all <p> elements that are descendants of the element with
id="main":
document.getElementById('main').getElementsByTagName("p"); |
DOM Node List
The getElementsByTagName() method
returns a node list. A node list is an array of nodes.
The following code stores a list
of <p> nodes (a node list) in the variable x:
x=document.getElementsByTagName("p"); |
The <p> elements in x can be
accessed by index number. To access the second <p> you can write:
y=x[1]; |
Note: The index starts at 0.
You will learn more about node
lists in a later chapter of this tutorial.
DOM Node List Length
The length property defines the
length of a node list (the number of nodes).
You can loop through a node list
by using the length property:
x=document.getElementsByTagName("p"); for (i=0;i<x.length;i++) { document.write(x[i].innerHTML); document.write("<br />"); } |
Example explained:
1. Get all
<p> element nodes
2. For each
<p> element, output the value of its text node
Navigating Node Relationships
The three properties parentNode,
firstChild, and lastChild follow the document structure and allow
short-distance travel in the document.
Look at the following HTML
fragment:
<html> <body> <p id="intro">W3Schools example</p> <div id="main"> <p id="main1">The DOM is very useful</p> <p id="main2">This example demonstrates <b>node relationships</b></p> </div> </body> </html> |
In the HTML code above, the <p
id="intro"> is the first child node (firstChild) of the
<body> element, and the <div> element is the last child node
(lastChild) of the <body> element.
Furthermore, the <body> is the
parent (parentNode) of the every <p> element.
The firstChild property can be
used to access the text of an element:
var x=document.getElementById("intro"); var text=x.firstChild.nodeValue; |
Root Nodes
There are two special document
properties that allow access to the tags:
- document.documentElement
- document.body
The first property returns the
root node of the document and exists in all XML and HTML documents.
The second property is a special
addition for HTML pages, and gives direct access to the <body> tag.
HTML DOM Node Information
The nodeName, nodeValue, and
nodeType properties contain information about nodes.
Node Properties
In the HTML Document Object Model
(DOM), each node is an object.
Objects have methods (functions)
and properties (information about the object), that can be accessed and
manipulated by JavaScript.
Three important HTML DOM node
properties are:
- nodeName
- nodeValue
- nodeType
The nodeName Property
The nodeName property specifies
the name of a node.
- nodeName is
read-only
- nodeName of an
element node is the same as the tag name
- nodeName of an
attribute node is the attribute name
- nodeName of a
text node is always #text
- nodeName of the
document node is always #document
Note: nodeName always contains the uppercase tag
name of an HTML element.
The nodeValue Property
The nodeValue property specifies
the value of a node.
- nodeValue for
element nodes is undefined
- nodeValue for
text nodes is the text itself
- nodeValue for
attribute nodes is the attribute value
Example1: Get the Value of an Element
The following code fragment
retrieves the text node value of the first <p> element:
x=document.getElementById("intro").firstChild; txt=x.nodeValue; |
Result: txt =
"W3Schools example"
Example explained:
1. Get text
node of the first <p> element node
2. Set the
txt variable to be the value of the text node
The nodeType Property
The nodeType property returns the
type of node.
nodeType is read only.
The most important node types are:
Element type
|
NodeType
|
Element
|
1
|
Attribute
|
2
|
Text
|
3
|
Comment
|
8
|
Document
|
9
|
HTML DOM - How to Change HTML
Elements
HTML elements are changed using
JavaScript, the HTML DOM and events.
Changing an HTML Element
The HTML DOM and JavaScript can be
used to change the inner content and attributes of HTML elements dynamically.
Example 1 - Change the Background Color
The following example changes the
background color of the <body> element:
<html> <body> <script type="text/javascript"> document.body.bgColor="yellow"; </script> </body> </html> |
Changing the Text HTML Element - innerHTML
The easiest way to get or modify
the content of an element is by using the innerHTML property.
Example 2 - Change the Text of an Element
The following example changes the
text of the <p> element:
<html> <body> <p id="p1">Hello World!</p> <script type="text/javascript"> document.getElementById("p1").innerHTML="New text!"; </script> </body> </html> |
Changing an HTML Element Using Events
An event handler allows you to
execute code when an event occurs.
Events are generated by the
browser when the user clicks an element, when the page loads, when a form is
submitted, etc.
You can read more about events in
the next chapter.
Example 3 - Change the Background Color
Using the onclick Event
The following example changes the
background color of the <body> element when the button is clicked:
<html> <body> <input type="button" onclick="document.body.bgColor='yellow';" value="Click me to change background color"> </body> </html> |
Example 4 - Create a Function to Change the
Text of an Element
The following example uses a
function to change the text of the <p> element when the button is
clicked:
<html> <head> <script type="text/javascript"> function ChangeText() { document.getElementById("p1").innerHTML="New text!"; } </script> </head> <body> <p id="p1">Hello world!</p> <input type="button" onclick="ChangeText()" value="Click me to change text above"> </body> </html> |
Using the Style Object
The Style object represents of
each HTML element represents its individual style.
The Style object can be accessed
from the document or from the elements to which that style is applied.
Example 5 - Change the Background Color
The following example uses a
function to change the style of the <body> element when the button is
clicked:
<html> <head> <script type="text/javascript"> function ChangeBackground() { document.body.style.backgroundColor="yellow"; } </script> </head> <body> <p id="p1">Hello world!</p> <input type="button" onclick="ChangeBackground()" value="Click me to change background color"> </body> </html> |
Example 6 - Change the Text font and color
of an Element
The following example uses a
function to change the style of the <p> element when the button is
clicked:
<html> <head> <script type="text/javascript"> function ChangeText() { document.getElementById("p1").style.color="blue"; document.getElementById("p1").style.fontFamily="Arial"; } </script> </head> <body> <p id="p1">Hello world!</p> <input type="button" onclick="ChangeText()" value="Click me to change text above"> </body> </html> |
HTML DOM Window Object
Window Object
The Window object is the top level
object in the JavaScript hierarchy.
The Window object represents a
browser window.
A Window object is created
automatically with every instance of a <body> or <frameset> tag.
IE: Internet Explorer, F: Firefox, O:
Opera.
Window Object Collections
Collection
|
Description
|
IE
|
F
|
O
|
frames[]
|
Returns all named frames in the window
|
4
|
1
|
9
|
Window Object Properties
Property
|
Description
|
IE
|
F
|
O
|
Returns whether or not a window has been closed
|
4
|
1
|
9
|
|
Sets or returns the default text in the statusbar of the window
|
4
|
No
|
9
|
|
4
|
1
|
9
|
||
4
|
1
|
9
|
||
length
|
Sets or returns the number of frames in the window
|
4
|
1
|
9
|
4
|
1
|
9
|
||
Sets or returns the name of the window
|
4
|
1
|
9
|
|
Returns a reference to the window that created the window
|
4
|
1
|
9
|
|
Sets or returns the outer height of a window
|
No
|
No
|
No
|
|
Sets or returns the outer width of a window
|
No
|
No
|
No
|
|
pageXOffset
|
Sets or returns the X position of the current page in relation
to the upper left corner of a window's display area
|
No
|
No
|
No
|
pageYOffset
|
Sets or returns the Y position of the current page in relation
to the upper left corner of a window's display area
|
No
|
No
|
No
|
parent
|
Returns the parent window
|
4
|
1
|
9
|
personalbar
|
Sets whether or not the browser's personal bar (or directories
bar) should be visible
|
|
|
|
scrollbars
|
Sets whether or not the scrollbars should be visible
|
|
|
|
Returns a reference to the current window
|
4
|
1
|
9
|
|
Sets the text in the statusbar of a window
|
4
|
No
|
9
|
|
statusbar
|
Sets whether or not the browser's statusbar should be visible
|
|
|
|
toolbar
|
Sets whether or not the browser's tool bar is visible or not
(can only be set before the window is opened and you must have
UniversalBrowserWrite privilege)
|
|
|
|
Returns the topmost ancestor window
|
4
|
1
|
9
|
Window Object Methods
Method
|
Description
|
IE
|
F
|
O
|
Displays an alert box with a message and an OK button
|
4
|
1
|
9
|
|
Removes focus from the current window
|
4
|
1
|
9
|
|
Cancels a timeout set with setInterval()
|
4
|
1
|
9
|
|
Cancels a timeout set with setTimeout()
|
4
|
1
|
9
|
|
Closes the current window
|
4
|
1
|
9
|
|
Displays a dialog box with a message and an OK and a Cancel
button
|
4
|
1
|
9
|
|
Creates a pop-up window
|
4
|
No
|
No
|
|
Sets focus to the current window
|
4
|
1
|
9
|
|
Moves a window relative to its current position
|
4
|
1
|
9
|
|
Moves a window to the specified position
|
4
|
1
|
9
|
|
Opens a new browser window
|
4
|
1
|
9
|
|
Prints the contents of the current window
|
5
|
1
|
9
|
|
Displays a dialog box that prompts the user for input
|
4
|
1
|
9
|
|
Resizes a window by the specified pixels
|
4
|
1
|
9
|
|
Resizes a window to the specified width and height
|
4
|
1.5
|
9
|
|
Scrolls the content by the specified number of pixels
|
4
|
1
|
9
|
|
Scrolls the content to the specified coordinates
|
4
|
1
|
9
|
|
Evaluates an expression at specified intervals
|
4
|
1
|
9
|
|
Evaluates an expression after a specified number of milliseconds
|
4
|
1
|
9
|
HTML DOM Navigator Object
Navigator Object
The Navigator object is actually a
JavaScript object, not an HTML DOM object.
The Navigator object is
automatically created by the JavaScript runtime engine and contains information
about the client browser.
IE: Internet Explorer, F: Firefox, O:
Opera.
Navigator Object Collections
Collection
|
Description
|
IE
|
F
|
O
|
plugins[]
|
Returns a reference to all embedded objects in the document
|
4
|
1
|
9
|
Navigator Object Properties
Property
|
Description
|
IE
|
F
|
O
|
Returns the code name of the browser
|
4
|
1
|
9
|
|
Returns the minor version of the browser
|
4
|
No
|
No
|
|
Returns the name of the browser
|
4
|
1
|
9
|
|
Returns the platform and version of the browser
|
4
|
1
|
9
|
|
Returns the current browser language
|
4
|
No
|
9
|
|
Returns a Boolean value that specifies whether cookies are
enabled in the browser
|
4
|
1
|
9
|
|
Returns the CPU class of the browser's system
|
4
|
No
|
No
|
|
Returns a Boolean value that specifies whether the system is in
offline mode
|
4
|
No
|
No
|
|
Returns the operating system platform
|
4
|
1
|
9
|
|
Returns the default language used by the OS
|
4
|
No
|
No
|
|
Returns the value of the user-agent header sent by the client to
the server
|
4
|
1
|
9
|
|
Returns the OS' natural language setting
|
4
|
No
|
9
|
Navigator Object Methods
Method
|
Description
|
IE
|
F
|
O
|
Specifies whether or not the browser has Java enabled
|
4
|
1
|
9
|
|
Specifies whether or not the browser has data tainting enabled
|
4
|
1
|
9
|
HTML DOM Document Object
Document Object
The Document object represents the
entire HTML document and can be used to access all elements in a page.
The Document object is part of the
Window object and is accessed through the window.document property.
IE: Internet Explorer, F: Firefox, O:
Opera, W3C: World Wide Web Consortium (Internet Standard).
Document Object Collections
Collection
|
Description
|
IE
|
F
|
O
|
W3C
|
Returns a reference to all Anchor objects in the document
|
4
|
1
|
9
|
Yes
|
|
Returns a reference to all Form objects in the document
|
4
|
1
|
9
|
Yes
|
|
Returns a reference to all Image objects in the document
|
4
|
1
|
9
|
Yes
|
|
Returns a reference to all Area and Link objects in the document
|
4
|
1
|
9
|
Yes
|
Document Object Properties
Property
|
Description
|
IE
|
F
|
O
|
W3C
|
body
|
Gives direct access to the <body> element
|
|
|
|
|
Sets or returns all cookies associated with the current document
|
4
|
1
|
9
|
Yes
|
|
Returns the domain name for the current document
|
4
|
1
|
9
|
Yes
|
|
Returns the date and time a document was last modified
|
4
|
1
|
No
|
No
|
|
Returns the URL of the document that loaded the current document
|
4
|
1
|
9
|
Yes
|
|
Returns the title of the current document
|
4
|
1
|
9
|
Yes
|
|
Returns the URL of the current document
|
4
|
1
|
9
|
Yes
|
Document Object Methods
Method
|
Description
|
IE
|
F
|
O
|
W3C
|
Closes an output stream opened with the document.open() method,
and displays the collected data
|
4
|
1
|
9
|
Yes
|
|
Returns a reference to the first object with the specified id
|
5
|
1
|
9
|
Yes
|
|
Returns a collection of objects with the specified name
|
5
|
1
|
9
|
Yes
|
|
Returns a collection of objects with the specified tagname
|
5
|
1
|
9
|
Yes
|
|
Opens a stream to collect the output from any document.write()
or document.writeln() methods
|
4
|
1
|
9
|
Yes
|
|
Writes HTML expressions or JavaScript code to a document
|
4
|
1
|
9
|
Yes
|
|
Identical to the write() method, with the addition of writing a
new line character after each expression
|
4
|
1
|
9
|
Yes
|
HTML DOM Form Object
Form Object
The Form object represents an HTML
form.
For each instance of a
<form> tag in an HTML document, a Form object is created.
IE: Internet Explorer, F: Firefox, O:
Opera, W3C: World Wide Web Consortium (Internet Standard).
Form Object Collections
Collection
|
Description
|
IE
|
F
|
O
|
W3C
|
Returns an array containing each element in the form
|
5
|
1
|
9
|
Yes
|
Form Object Properties
Property
|
Description
|
IE
|
F
|
O
|
W3C
|
Sets or returns a list of possible character-sets for the form
data
|
No
|
No
|
No
|
Yes
|
|
Sets or returns the action attribute of a form
|
5
|
1
|
9
|
Yes
|
|
Sets or returns the MIME type used to encode the content of a
form
|
6
|
1
|
9
|
Yes
|
|
Sets or returns the id of a form
|
5
|
1
|
9
|
Yes
|
|
Returns the number of elements in a form
|
5
|
1
|
9
|
Yes
|
|
Sets or returns the HTTP method for sending data to the server
|
5
|
1
|
9
|
Yes
|
|
Sets or returns the name of a form
|
5
|
1
|
9
|
Yes
|
|
Sets or returns where to open the action-URL in a form
|
5
|
1
|
9
|
Yes
|
Standard Properties
Property
|
Description
|
IE
|
F
|
O
|
W3C
|
Sets or returns the class attribute of an element
|
5
|
1
|
9
|
Yes
|
|
Sets or returns the direction of text
|
5
|
1
|
9
|
Yes
|
|
Sets or returns the language code for an element
|
5
|
1
|
9
|
Yes
|
|
Sets or returns an element's advisory title
|
5
|
1
|
9
|
Yes
|
Form Object Methods
Method
|
Description
|
IE
|
F
|
O
|
W3C
|
Resets the values of all elements in a form
|
5
|
1
|
9
|
Yes
|
|
Submits a form
|
5
|
1
|
9
|
Yes
|
HTML DOM Button Object
Button Object
The Button object represents a
push button.
For each instance of a
<button> tag in an HTML document, a Button object is created.
IE: Internet Explorer, F: Firefox, O:
Opera, W3C: World Wide Web Consortium (Internet Standard).
Button Object Properties
Property
|
Description
|
IE
|
F
|
O
|
W3C
|
Sets or returns the keyboard key to access a button
|
6
|
1
|
9
|
Yes
|
|
Sets or returns whether a button should be disabled
|
6
|
1
|
9
|
Yes
|
|
Returns a reference to the form that contains the button
|
6
|
1
|
9
|
Yes
|
|
Sets or returns the id of a button
|
6
|
1
|
9
|
Yes
|
|
Sets or returns the name of a button
|
6
|
1
|
9
|
Yes
|
|
Sets or returns the tab order for a button
|
6
|
1
|
9
|
Yes
|
|
Returns the type of form element a button is
|
6
|
1
|
9
|
Yes
|
|
Sets or returns the text that is displayed on a button
|
6
|
1
|
9
|
Yes
|
Standard Properties
Property
|
Description
|
IE
|
F
|
O
|
W3C
|
Sets or returns the class attribute of an element
|
5
|
1
|
9
|
Yes
|
|
Sets or returns the direction of text
|
5
|
1
|
9
|
Yes
|
|
Sets or returns the language code for an element
|
5
|
1
|
9
|
Yes
|
|
Sets or returns an element's advisory title
|
5
|
1
|
9
|
Yes
|
HTML DOM Text Object
Text Object
The Text object represents a
text-input field in an HTML form.
For each instance of an <input
type="text"> tag in an HTML form, a Text object is created.
You can access a text-input field
by searching through the elements[] array of the form, or by using
document.getElementById().
IE: Internet Explorer, F: Firefox, O:
Opera, W3C: World Wide Web Consortium (Internet Standard).
Text Object Properties
Property
|
Description
|
IE
|
F
|
O
|
W3C
|
Sets or returns the keyboard key to access a text field
|
4
|
1
|
9
|
Yes
|
|
Sets or returns an alternate text to display if a browser does
not support text fields
|
5
|
1
|
9
|
Yes
|
|
Sets or returns the default value of a text field
|
4
|
1
|
9
|
Yes
|
|
Sets or returns whether or not a text field should be disabled
|
5
|
1
|
9
|
Yes
|
|
Returns a reference to the form that contains the text field
|
4
|
1
|
9
|
Yes
|
|
Sets or returns the id of a text field
|
4
|
1
|
9
|
Yes
|
|
Sets or returns the maximum number of characters in a text field
|
4
|
1
|
9
|
Yes
|
|
Sets or returns the name of a text field
|
4
|
1
|
9
|
Yes
|
|
Sets or returns whether or not a text field should be read-only
|
4
|
1
|
9
|
Yes
|
|
Sets or returns the size of a text field
|
4
|
1
|
9
|
Yes
|
|
Sets or returns the tab order for a text field
|
4
|
1
|
9
|
Yes
|
|
Returns the type of form element a text field is
|
4
|
1
|
9
|
Yes
|
|
Sets or returns the value of the value attribute of a text field
|
4
|
1
|
9
|
Yes
|
Standard Properties
Property
|
Description
|
IE
|
F
|
O
|
W3C
|
Sets or returns the class attribute of an element
|
5
|
1
|
9
|
Yes
|
|
Sets or returns the direction of text
|
5
|
1
|
9
|
Yes
|
|
Sets or returns the language code for an element
|
5
|
1
|
9
|
Yes
|
|
Sets or returns an element's advisory title
|
5
|
1
|
9
|
Yes
|
Text Object Methods
Method
|
Description
|
IE
|
F
|
O
|
W3C
|
Removes focus from a text field
|
4
|
1
|
9
|
Yes
|
|
Sets focus on a text field
|
4
|
1
|
9
|
Yes
|
|
Selects the content of a text field
|
4
|
1
|
9
|
Yes
|
Introduction to DHTML
DHTML is the art of combining
HTML, JavaScript, DOM, and CSS.
What you should already know
Before you continue you should
have a basic understanding of the following:
- HTML
- JavaScript
- CSS
If you want to study these
subjects first, find the tutorials on our Home Page.
DHTML is Not a Language
DHTML stands for Dynamic HTML.
DHTML is NOT a language or a web
standard.
DHTML is a TERM used to describe
the technologies used to make web pages dynamic and interactive.
To most people DHTML means the
combination of HTML, JavaScript, DOM, and CSS.
According to the World Wide Web
Consortium (W3C):
"Dynamic HTML is a term used by some vendors to describe the
combination of HTML, style sheets and scripts that allows documents to be
animated."
DHTML Technologies
Below is a listing of DHTML technologies.
HTML 4
The W3C HTML 4 standard has rich
support for dynamic content:
- HTML supports
JavaScript
- HTML supports
the Document Object Model (DOM)
- HTML supports
HTML Events
- HTML supports
Cascading Style Sheets (CSS)
DHTML is about using these
features to create dynamic and interactive web pages.
JavaScript
JavaScript is the scripting
standard for HTML.
DHTML is about using JavaScript to
control, access and manipulate HTML elements.
You can read more about this in
the next chapter of this tutorial.
HTML DOM
The HTML DOM is the W3C standard Document
Object Model for HTML.
The HTML DOM defines a standard
set of objects for HTML, and a standard way to access and manipulate them.
DHTML is about using the DOM to
access and manipulate HTML elements.
You can read more about this in a
later chapter of this tutorial.
HTML Events
The W3C HTML Event Model is a part
of the HTML DOM.
It defines a standard way to
handle HTML events.
DHTML is about creating web pages
that reacts to (user)events.
You can read more about this in a
later chapter of this tutorial.
CSS
CSS is the W3C standard style and
layout model for HTML.
CSS allows web developers to
control the style and layout of web pages.
HTML 4 allows dynamic changes to
CSS.
DHTML is about using JavaScript
and DOM to change the style and positioning of HTML elements.
You can read more about this in a
later chapter of this tutorial.
DHTML JavaScript
JavaScript can create dynamic HTML
content:
Date: Thu Jul 31 13:47:42 2008
JavaScript Alone
If you have studied JavaScript,
you already know that the statement:
document.write()
can be used to display dynamic
content to a web page.
Example
Using JavaScript to display the
current date:
<html> <body> <script type="text/javascript"> document.write(Date()); </script> </body> </html> |
JavaScript and the HTML DOM
With HTML 4, JavaScript can also
be used to change the inner content and attributes of HTML elements
dynamically.
To change the content of an HTML
element use:
document.getElementById(id).innerHTML=new HTML
To change the attribute of an HTML
element use:
document.getElementById(id).attribute=new
value
You will learn more about
JavaScript and the HTML DOM in the next chapter of this tutorial.
JavaScript and HTML Events
New to HTML 4 is the ability to
let HTML events trigger actions in the browser, like starting a JavaScript when
a user clicks on an HTML element.
To execute code when a user clicks
on an element, use the following event attribute:
onclick=JavaScript
You will learn more about
JavaScript and HTML Events in a later chapter.
JavaScript and CSS
With HTML 4, JavaScript can also
be used to change the style of HTML elements.
To change the style of an HTML
element use:
document.getElementById(id).style.property=new style
You will learn more about
JavaScript and CSS in a later chapter of this tutoria
DHTML - HTML Document Object
Model (DOM)
The DOM presents HTML as a
tree-structure (a node tree), with elements, attributes, and text:

Examples
How to access and change the innerHTML of an element.
How to access an image element and change the "src" attribute.
What is the HTML DOM?
The HTML DOM is:
- A standard object model for HTML
- A standard programming interface
for HTML
- Platform- and
language-independent
- A W3C standard
The HTML DOM defines the objects
and properties of all HTML elements, and the methods (interface) to
access them.
In other words:
The HTML DOM is a standard for
how to get, change, add, or delete HTML elements.
Using the HTML DOM to Change an HTML Element
The HTML DOM can be used to change
the content of an HTML element:
<html> <body> <h1 id="header">Old Header</h1> <script type="text/javascript"> document.getElementById("header").innerHTML="New Header"; </script> </body> </html> |
HTML output:
New Header
|
Example explained:
- The HTML
document contains a header with id="header"
- The DOM is used
to get the element with id="header"
- A JavaScript is
used to change the HTML content (innerHTML)
Using the HTML DOM to Change an HTML
Attribute
The HTML DOM can be used to change
the attribute of an HTML element:
<html> <body> <img id="image" src="smiley.gif"> <script type="text/javascript"> document.getElementById("image").src="landscape.jpg"; </script> </body> </html> |
HTML output:
![]() |
Example explained:
- The HTML document loads with an
image with id="image"
- The DOM is used to get the
element with id="image"
- A JavaScript changes the scr
attribute from smiley.gif to landscape.jpg
More About the HTML DOM
If you want to study more about
the HTML DOM, find the complete HTML DOM tutorial on our Home Page.
HTML 4.0 Event Handlers
Event
|
Occurs when...
|
onabort
|
a user aborts page loading
|
onblur
|
a user leaves an object
|
onchange
|
a user changes the value of an object
|
onclick
|
a user clicks on an object
|
ondblclick
|
a user double-clicks on an object
|
onfocus
|
a user makes an object active
|
onkeydown
|
a keyboard key is on its way down
|
onkeypress
|
a keyboard key is pressed
|
onkeyup
|
a keyboard key is released
|
onload
|
a page is finished loading
|
onmousedown
|
a user presses a mouse-button
|
onmousemove
|
a cursor moves on an object
|
onmouseover
|
a cursor moves over an object
|
onmouseout
|
a cursor moves off an object
|
onmouseup
|
a user releases a mouse-button
|
onreset
|
a user resets a form
|
onselect
|
a user selects content on a page
|
onsubmit
|
a user submits a form
|
onunload
|
a user closes a page
|
DHTML CSS
JavaScript can be used to change
the style an HTML element:
Examples
How to change the style of an element using the this object.
How to change the style of an element using getElementById.
(You can find more examples at the
bottom of this page)
JavaScript, DOM, and CSS
With HTML 4, JavaScript and the
HTML DOM can be used to change the style any HTML element.
To change the style the current HTML element, use the statement:
style.property=new style
or more correctly:
this.style.property=new
style
To change the style of a specific
HTML element, use the statement:
document.getElementById(element_id).style.property=new style
More examples
How to change the color of an element when the cursor moves over it.
How to make an element invisible. Do you want the element to show or not?
More About JavaScript, DOM, And CSS
For a full overview please refer
to the complete DOM Style Object Reference in our HTML DOM tutorial.
To learn more about CSS, find the
complete CSS tutorial on our Home
Page.
Function
|
Start Tag
|
Attributes
|
End Tag
|
HTML File
|
<html>
|
none
|
</html>
|
File Header
|
<head>
|
none
|
</head>
|
File Title
|
<title>
|
none
|
</title>
|
Comments
|
<!--
|
Your comments go between the
start and end tags. Put a space between the -- and your comments.
|
-->
|
Body
|
<body>
|
background="filename"
bgcolor="color value" text="color value" link="color value" vlink="color value" |
</body>
|
Division
|
<div>
|
align="right/left/center"
style="property:value;" class="classname" |
</div>
|
Span (inline)
|
<span>
|
style="property:value;"
class="classname" |
</span>
|
Basic Text Tags
|
|||
Function
|
Start Tag
|
Attributes
|
End Tag
|
Line Break
|
<br>
|
clear="left/right/all"
|
</br> or <br />
|
Paragraph
|
<p>
|
align="center/right"
|
</p>
|
Bold
|
<b>
|
none
|
</b>
|
Italic
|
<i>
|
none
|
</i>
|
Typewriter Text
|
<tt>
|
none
|
</tt>
|
Headline
|
<h1-6>
|
align="center/right"
|
</h1-6>
|
Font
|
<font>
|
face="name, name"
size="+/-value/fixed size" color="color value" Note: the font tag is being phased out in favor of CSS styles. |
</font>
|
Horizontal Rule
|
<hr>
|
size="XX"
width="XX/XX%" noshade |
</hr> or <hr />
|
Block Quote
|
<blockquote>
|
none
|
</blockquote>
|
Division
|
<div>
|
align="left/center/right"
|
</div>
|
List Tags
|
|||
Function
|
Start Tag
|
Attributes
|
End Tag
|
Unordered List
|
<ul>
|
type="disc/circle/square"
|
</ul>
|
Ordered List
|
<ol>
|
type="I/A/1/a/i"
start="value to start counting at" |
</ol>
|
List Item
|
<li>
|
type=all ul and ol options
|
</li>
|
Definiton List
|
<dl>
|
none
|
</dl>
|
Definition List Item
|
<dt>
|
none
|
</dt>
|
Definition List Definition
|
<dd>
|
none
|
</dd>
|
Link Tags
|
|||
|
|
|
|
Function
|
Start Tag
|
Attributes
|
End Tag
|
Anchor Link
|
<a>
|
href="filename"
target="windowname" |
</a>
|
Anchor Mark
|
<a>
|
name="markname"
|
</a>
|
|
|
|
|
Image Tags
|
|||
Function
|
Start Tag
|
Attributes
|
End Tag
|
Insert Image
|
<img>
|
src="filename"
align="left/right" width="XXX" height="XXX" alt="text that desribes image" ISMAP USEMAP="#mapname" |
</img>
|
Client-side Imagemap Tags
|
|||
Function
|
Start Tag
|
Attributes
|
End Tag
|
Define Map
|
<map>
|
name="mapname"
|
</map>
|
Area Definition
|
<area>
|
shape="rect/circle/poly/point"
coords="X,Y,X,Y" href="imagename" |
</area>
|
Table Tags
|
|||
Function
|
Start Tag
|
Attributes
|
End Tag
|
Table
|
<table>
|
border="X"
width="XX/X%" cellspacing="XX" cellpadding="XX" bgcolor="color value" background="filename" |
</table>
|
Table Row
|
<tr>
|
align="left/center/right"
valign="top/middle/bottom" bgcolor="color value" |
</tr>
|
Table Data
|
<td>
|
align="left/center/right"
valign="top/middle/bottom" width=X nowrap colspan="X" rowspan="X" bgcolor="color value" |
</td>
|
Table Header
|
<th>
|
align="left/center/right"
valign="top/middle/bottom" width=X nowrap colspan="X" rowspan="X" bgcolor="color value" |
</th>
|
Caption
|
<caption>
|
align="left/center/right"
valign="top/middle/bottom" |
</caption>
|
Frame Tags
|
|||
Function
|
Start Tag
|
Attributes
|
End Tag
|
Set Frames
|
<frameset>
|
cols="XX/XX%/*"
rows="XX/XX%/*" |
</frameset>
|
Frame Definition
|
<frame>
|
src="filename"
name="framename" noresize scroll=auto/yes/no marginwidth="XX" marginheight="XX" |
</frame>
|
Base
|
<base>
|
target="framename"/
"_self"/ "_top"/ "_parent" (Note the underscores) |
</base>
|
No Frames
|
<noframes>
|
Between start and end tags, place the content that appears when
a non-frames browser loads this page.
|
</noframes>
|
Form Tags
|
|||
Function
|
Start Tag
|
Attributes
|
End Tag
|
Form
|
<form>
|
method=get/put
action="programname" |
</form>
|
Input Field
|
<input>
|
name="variablename"
type=text/password/ checkbox/radio/submit/ reset/image |
</input>
|
Selection List
|
<select>
|
name="variablename"
size=XX multiple |
</select>
|
Selection Option
|
<option>
|
none
|
</option>
|
Scrolling Text Field
|
<textarea>
|
name="variablename"
rows=XX cols=XX |
</textarea>
|
|
|||
Function
|
Start Tag
|
Attributes
|
End Tag
|
<meta>
(Server metatags) |
http-equiv="refresh"
content="seconds, filename" |
none
|
</meta>
|
<meta>
(Content metatags) |
name="keywords/description/author/
generator/abstract/expiration" content="your information" |
none
|
</meta>
|
Style Sheet Tags
|
|||
Function
|
Start Tag
|
Attributes
|
End Tag
|
Style Definition Area
|
<style>
|
type="text/css">
Style declarations go between begin and end style tags |
</style>
|
Link to external CSS File
|
<link>
|
rel=stylesheet
type="text/css" href="URL" |
none
|
Commonly-Used Special Characters
|
||
Name
|
Symbol
|
HTML Equivalent
|
ampersand
|
&
|
&
|
cent sign
|
¢
|
¢
|
copyright symbol
|
©
|
© or ©
|
degree sign
|
°
|
°
|
greater than
|
>
|
>
|
less than
|
<
|
<
|
non-breaking space
|
|
|
registered trademark
|
®
|
®
|
trademark
|
|
™
|
0 comments:
Post a Comment